We’re already discussed the value of including version information in your binaries. Last post we talked about Versioning a Native C/C++ Binary with Visual Studio. This time we are going to talk about how to do this with a .NET assembly with Visual Studio. I’ll be using C# and Visual Studio 2010 but the principals work in VB .NET and other versions of Visual Studio as well.
First of all, credit where credit is due. Many of the details below are based on this stackoverflow question.
The source code for this post is available here.
Step 1: It’s Already Done!
Whenever you create a new .NET project in Visual Studio a file named AssemblyInfo.cs is created for you under the Properties folder. The annotations in the AssemblyInfo.cs file define the version characteristics include those found in the file properties. By default you’ll get a file like this which sets the copyright to Microsoft, the version to a fixed 1.0.0.0 (more on that in a moment) and a title that matches the project name.
using System.Reflection; using System.Runtime.CompilerServices; using System.Runtime.InteropServices; // General Information about an assembly is controlled through the following // set of attributes. Change these attribute values to modify the information // associated with an assembly. [assembly: AssemblyTitle("ClassLibrary1")] [assembly: AssemblyDescription("")] [assembly: AssemblyConfiguration("")] [assembly: AssemblyCompany("Microsoft")] [assembly: AssemblyProduct("ClassLibrary1")] [assembly: AssemblyCopyright("Copyright © Microsoft 2011")] [assembly: AssemblyTrademark("")] [assembly: AssemblyCulture("")] // Setting ComVisible to false makes the types in this assembly not visible // to COM components. If you need to access a type in this assembly from // COM, set the ComVisible attribute to true on that type. [assembly: ComVisible(false)] // The following GUID is for the ID of the typelib if this project is exposed to COM [assembly: Guid("6e0a5113-8d8a-4177-8de4-1c18b5b2c446")] // Version information for an assembly consists of the following four values: // // Major Version // Minor Version // Build Number // Revision // // You can specify all the values or you can default the Build and Revision Numbers // by using the '*' as shown below: // [assembly: AssemblyVersion("1.0.*")] [assembly: AssemblyVersion("1.0.0.0")] [assembly: AssemblyFileVersion("1.0.0.0")]
You can make changes directly to this file however after you have several projects in the same solution, it can get rather tedious to manually update this duplicative information across all your assemblies.
Step 2: Add a Solution-wide GlobalAssemblyInfo File for Common Info
To make life a little simpler moving forward and in keeping with that whole DRY thing you can use a single file to hold all the assembly information that is common across multiple projects. I generally organize my source tree such that there is a top-level Code directory, with separate sub-directories for each project. Therefore I find it most convenient to place a file named GlobalAssemblyInfo.cs in the Code directory, peer to all my project directories. You can place the file wherever you want, however.
Place common version info inside such as:
// This file contains common AssemblyVersion data to be shared across all projects in this solution. using System.Reflection; [assembly: AssemblyCompany("Zach Burlingame")] [assembly: AssemblyProduct("DotNetVersionAssembly")] [assembly: AssemblyCopyright("Copyright © Zach Burlingame 2011")] [assembly: AssemblyTrademark("")] [assembly: AssemblyCulture("")] #if DEBUG [assembly: AssemblyConfiguration("Debug")] #else [assembly: AssemblyConfiguration("Release")] #endif // Version information for an assembly consists of the following four values: // // Major Version // Minor Version // Revision // Build // // You can specify all the values or you can default the Revision and Build Numbers by using the '*' as shown below: // [assembly: AssemblyVersion("1.0.*")] [assembly: AssemblyVersion("1.0.0.*")] //[assembly: AssemblyFileVersion("1.0.0.*")]
Step 3: Add a link to the GlobalAssemblyInfo.cs file in each project
- In Visual Studio, right-click on a project
- Select Add->Existing Item
- Browse to GlobalAssemblyInfo.cs
- Select the file
- Click the drop-down arrow next to Add and select Add As Link
- Move the link to your Properties folder (optional, but keeps things neat)
Step 4: Update the AssemblyInfo.cs for each project
In order to avoid duplicate annotations for assembly information, you must remove entries from the AssemblyInfo.cs file that appear in the GlobalAssemblyInfo.cs file. In our example here, this is what we end up with:
using System.Reflection; using System.Runtime.CompilerServices; using System.Runtime.InteropServices; // General Information about an assembly is controlled through the following // set of attributes. Change these attribute values to modify the information // associated with an assembly. [assembly: AssemblyTitle("DotNetVersionAssembly")] [assembly: AssemblyDescription("")] // Setting ComVisible to false makes the types in this assembly not visible // to COM components. If you need to access a type in this assembly from // COM, set the ComVisible attribute to true on that type. [assembly: ComVisible(false)] // The following GUID is for the ID of the typelib if this project is exposed to COM [assembly: Guid("dad09178-814d-43f4-b76d-0fbe29a32544")]
Step 5: Auto-incrementing the build number
You might of noticed a couple of other changes I made in Step 3:
// Version information for an assembly consists of the following four values: // // Major Version // Minor Version // Revision // Build // // You can specify all the values or you can default the Revision and Build Numbers by using the '*' as shown below: // [assembly: AssemblyVersion("1.0.*")] [assembly: AssemblyVersion("1.0.0.*")] //[assembly: AssemblyFileVersion("1.0.0.*")]
By replacing build value with an asterisk, Visual Studio (and more specifically, MSBuild) will auto-increment that number every time you build. If you replace both the revision and the build value with a single asterisk it will cause both the revision and build number to auto-increment. This is helpful since the maximum value of any single field is 65535. By auto-incrementing both fields, the revision field is only incremented when the build value overflows, giving you in effect 4 billion unique version numbers for a given Major.Minor combination. If you overflow that, then for the love of pete increment your Major version already!
Also notice that I commented out the AssemblyFileVersion. The differences between the AssemblyVersion and AssemblyFileVersion are discussed here. The key in our example is that you can’t use asterisks to auto-increment the AssemblyFileVersion like you can the AssemblyVersion and you probably don’t want the two getting out-of-sync (at least in this versioning scheme). By not providing an AssemblyFileVersion, MSBuild will default to using the value from the AssemblyVersion.
Results
Now when you build your application, all the version info above will be defined in the assembly itself. Much of this information is available directly from the Details pane of the Properties window in Explorer.
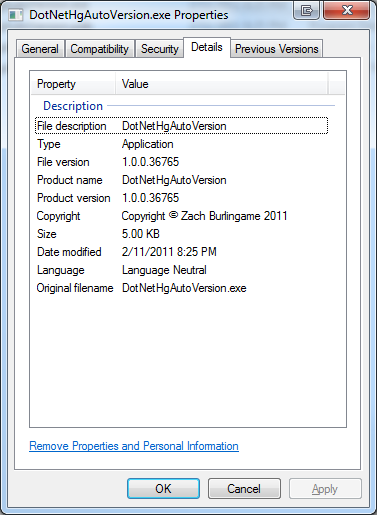
Final Thoughts
Note that while it is possible to auto-increment the build and revision number of the assemblies, those numbers don’t correspond to anything in source control. I’ll be discussing how to make the BUILD number automatically correspond to the revision info from the working copy of the source code using Mercurial or Subversion in an upcoming post.
Other Posts in this Series
- Mapping Binaries in the Field to Source Code in the Repository
- Versioning a Native C/C++ Binary with Visual Studio
- Versioning a .NET Assembly with Visual Studio
- Integrating the Mercurial Revision into the Version Automatically with Native C/C++
- Integrating the Mercurial Revision into the Version Automatically with .NET
- Integrating the Subversion Revision into the Version Automatically with Native C/C++
- Integrating the Subversion Revision into the Version Automatically with .NET
Leave a Reply