Now that we’ve covered why we should include version information in our binaries and how to do that for native C/C++ and managed .NET projects, it’s time to up our game. One of the major shortfalls of the previous solutions is that the version information on the binary didn’t map one-to-one to revisions from source control. As a result, while you may be able to determine the version of a particular file, you can’t easily get to the corresponding source code, if at all. One solution to this is to include the source control revision information in the version so you know exactly what was used to build it. Here I’ll be building on the previous posts and discussing how to do this with Visual Studio 2010 Professional and Mercurial using TortoiseHg for a native C/C++ application. Other versions of Visual Studio should work similarly and other Mercurial packages will work as well, as long as they provide command-line tools that are in the path of your IDE. The steps can be easily modified for use with a .NET project based on the previous post.
Update 2011/02/17: Doing this for a .NET assembly is actually a bit trickier than I originally thought because you can’t use static variables or class data in the assembly attributes – you have to use constant literals or expressions. I’ll do a separate post on how to do this with a .NET project.
The basic concept of how this works is explained in the here. The source code for this post is available here.
Step 1: Using WSH to Generate a Version Header
The first step is to add a file to your project named hg_version.jse. Personally, I add this file under the Resource filter of my project. Copy the code below in to the script file. This code does two things:
- Creates hg_version.h
- Extracts the desired Mercurial version info from the working copy and places it in the header
The extracted version info includes the full node identity, the short node identity, the revision number, and if there are any local modifications to the working copy.
var fso = new ActiveXObject("Scripting.FileSystemObject"); var shell = new ActiveXObject("WScript.Shell"); var outFile = fso.CreateTextFile("hg_version.h", true); var hgRevNum = shell.Exec("hg identify --num"); var rev = hgRevNum.StdOut.ReadAll(); var hg_revision = String(rev).replace(/\n/g,"").replace(/\+/g,""); var hg_local_modifications = 0 if( String(rev).replace(/\n/g, "").indexOf("+") != -1 ) { hg_local_modifications = 1; } outFile.WriteLine( "#define HG_REVISION " + hg_revision ); outFile.WriteLine( "#define HG_LOCAL_MODIFICATIONS " + hg_local_modifications ); var hgChangeset = shell.Exec("hg parents --template \"{node}\""); var changeset = hgChangeset.StdOut.ReadAll(); var hg_changeset = String(changeset).replace(/\n/g,""); outFile.WriteLine( "#define HG_CHANGESET \"" + hg_changeset +"\"" ); var hgChangesetShort = shell.Exec("hg parents --template \"{node|short}\""); var changeset_short = hgChangesetShort.StdOut.ReadAll(); var hg_changeset_short = "#define HG_CHANGESET_SHORT \"" + String(changeset_short).replace(/\n/g,"") + "\""; outFile.WriteLine( hg_changeset_short ); outFile.Close();
Step 2: Update Version.h
The version.h file we created in a previous post needs to be updated to use the information from the generated header. The mercurial revision number is a human friendly integer and can be used directly in the file version. However, due to the nature of a DVCS it is not guaranteed to be globally unique (and it often won’t be on projects with multiple developers using common workflow patterns). The node identity however, does uniquely identify the changeset globally. We include this information in the file description field which maps the binaries one-to-one with the source code they were built with. It’s important to note that the identity field is not a 16-bit integer and thus cannot be used in the file version field directly. Finally, we want to know if the binary was built with local modifications to the working copy, which would complicate reproducing the build. As a result we append an ‘M’ to the end of the file version string if local modifications are present.
#include "hg_version.h" #define STRINGIZE2(s) #s #define STRINGIZE(s) STRINGIZE2(s) #define VERSION_MAJOR 1 #define VERSION_MINOR 0 #define VERSION_REVISION 0 #define VERSION_BUILD HG_REVISION #if HG_LOCAL_MODIFICATIONS #define VERSION_MODIFIER "M" #else #define VERSION_MODIFIER #endif #define VER_FILE_DESCRIPTION_STR HG_CHANGESET #define VER_FILE_VERSION VERSION_MAJOR, VERSION_MINOR, VERSION_REVISION, VERSION_BUILD #define VER_FILE_VERSION_STR STRINGIZE(VERSION_MAJOR) \ "." STRINGIZE(VERSION_MINOR) \ "." STRINGIZE(VERSION_REVISION) \ "." STRINGIZE(VERSION_BUILD) \ VERSION_MODIFIER #define VER_PRODUCTNAME_STR "c_hg_autoversion" #define VER_PRODUCT_VERSION VER_FILE_VERSION #define VER_PRODUCT_VERSION_STR VER_FILE_VERSION_STR #if LIBRARY_EXPORTS #define VER_ORIGINAL_FILENAME_STR VER_PRODUCTNAME_STR ".dll" #else #define VER_ORIGINAL_FILENAME_STR VER_PRODUCTNAME_STR ".exe" #endif #define VER_INTERNAL_NAME_STR VER_ORIGINAL_FILENAME_STR #define VER_COPYRIGHT_STR "Copyright (C) 2011" #ifdef _DEBUG #define VER_VER_DEBUG VS_FF_DEBUG #else #define VER_VER_DEBUG 0 #endif #define VER_FILEOS VOS_NT_WINDOWS32 #define VER_FILEFLAGS VER_VER_DEBUG #if LIBRARY_EXPORTS #define VER_FILETYPE VFT_DLL #else #define VER_FILETYPE VFT_APP #endif
Step 3: Add the Pre-build Step
Finally we need to add a pre-build step which will execute the hg_version.jse script, thus generating the hg_version.h file prior to the binary being built.
- Right-click on your project
- Select Properties
- Click Build Events
- Click Pre-Build Event
- In the Configuration drop-down, select All Configurations
- In the Command Line field, enter:
cscript.exe hg_version.jse - In the Description field, add a comment such as:
Generate the hg_version.h file with the necessary repo identify info for versioning
NOTE:If you have multiple projects in the same solution that all need to use the same information from Mercurial you have a few choices. One is to put the hg_version.jse script in one project which all the other projects depend on and add a link to the hg_version.h file. Another option is to create a specific version project that all it does is generate the hg_version.h file and define common version information and then have every project in the solution depends on it so it’s executed first in the build order.
Results
Now when each time you build your projects, the latest Mercurial information of the working copy is automatically included in the file version information.
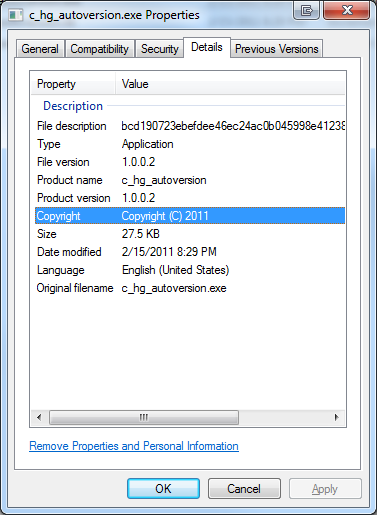
Final Thoughts
So there you have it, you can now automatically include all the necessary information from your Mercurial working copy in your build to map them one-to-one with the source code that was used. In an upcoming post I’ll discuss how to do this using Subversion as your VCS.
Other Posts in this Series
- Mapping Binaries in the Field to Source Code in the Repository
- Versioning a Native C/C++ Binary with Visual Studio
- Versioning a .NET Assembly with Visual Studio
- Integrating the Mercurial Revision into the Version Automatically with Native C/C++
- Integrating the Mercurial Revision into the Version Automatically with .NET
- Integrating the Subversion Revision into the Version Automatically with Native C/C++
- Integrating the Subversion Revision into the Version Automatically with .NET
Leave a Reply